Auth0
Overview
Auth0 is a highly adaptable authentication and authorization platform. Median's Auth0 Native Plugin implements the Auth0 iOS and Android SDK into your app.
The Auth0 integration allows you to request a login token from Auth0 using Universal Login and a native login UI. And optionally to store a refresh token to the secure device storage so that future logins are seamless using Face ID / Touch ID and Android Biometric. This biometric functionality is similar to our Face ID / Touch ID Android Biometric Native Plugin.
Developer Demo
Display our demo page in your app to test during development https://median.dev/auth0
Implementation Guide
Configuration
The Auth0 Native Plugin requires several parameters to be set in the App Studio, on the Native Plugins tab under "Advanced Mode". Sample parameters are as follows:
{
"domain": "median-test.auth0.com",
"clientId": "q6jYUAy9e0c3MPOryIthwldSAAQFQhI",
"scheme": "co.median.median-test",
"audience":"https://median-test.auth0.com/api/v2/" // Optional based on Auth0 tenant config
}
These parameters should match the Application Settings on your Auth0 Dashboard.
Additionally you will need to verify that Deep Linking is configured properly for all domains listed under Universal Links / Deep Links and that a URL Scheme Protocol is specified for your app. The URL Scheme and all other values including Package Name and Bundle ID must match the parameters set in your Auth0 tenant.
Login using Universal Login
Launch Universal Login via the JavaScript Bridge. Upon completion of Universal Login flow focus is returned to the app webview and a promise/callback function is invoked with the tokens as parameters.
↔️Median JavaScript Bridge
To prompt Universal Login and retrieve a token:
const credentials = await median.auth0.login( { scope: "email profile offline_access" // optionally pass a scope } ); // credentials object will have the following structure { accessToken: STRING, idToken: STRING, scope: STRING, error: STRING, // if an error occurred, refreshToken: STRING // with 'offline_access' scope configured }
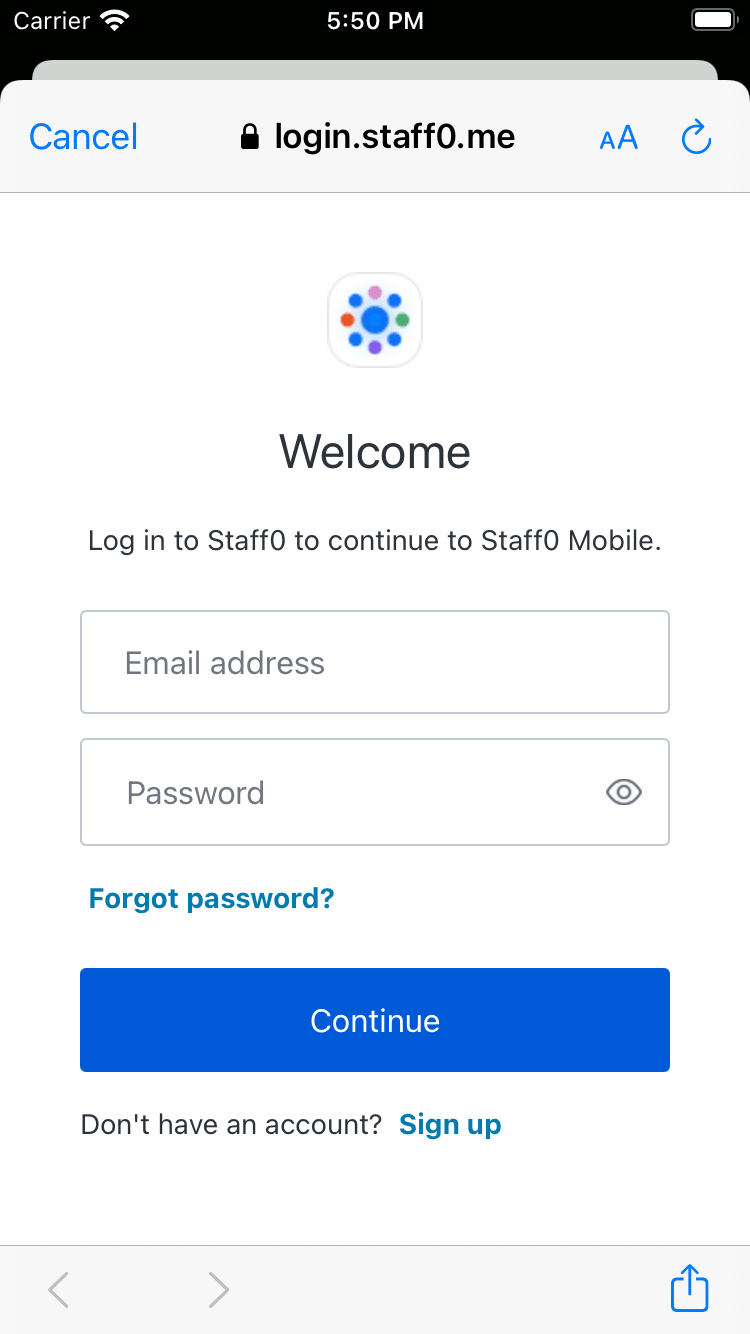
Auth0 Universal Login
Logout
↔️Median JavaScript Bridge
To logout:
median.auth0.logout.then(function(){ error: STRING // if an error occurred }
Support for Face ID / Touch ID / Android Biometrics
↔️Median JavaScript Bridge
// you can enable support for Face ID / Touch ID / Android Biometrics when logging in. median.auth0.login({ enableBiometrics: true, callback: median_auth0_post_login }); // To find out if biometrics were enabled upon login const auth0Status = await median.auth0.status(); // status object will have the following structure { biometryAvailable: boolean, biometryType: 'touchId' | 'faceId' | 'none', hasValidCredentials: boolean }
Get saved credentials
Credentials are saved in the secure storage and can be retrieved in later sessions. This will automatically renew the credentials using the refreshToken
already saved from prior successful login
↔️Median JavaScript Bridge
To get credentials saved in secure storage
const credentials = await median.auth0.getCredentials(); // credentials object will have the following structure { accessToken: STRING, idToken: STRING, scope: STRING, error: STRING, // if an error occurred, refreshToken: STRING }
Renew
Retrieving the credentials gets you renewed credentials if existing ones are expired but you can also request to renew the credentials manually as well.
You can optionally pass a refresh token to be used. By default it will use the one saved in credentials.
↔️Median JavaScript Bridge
To logout:
const credentials median.auth0.renew({ // optionally pass a refresh token refreshToken?: string });
Updated 2 months ago